Firebase Login and Authentication: Android Tutorial
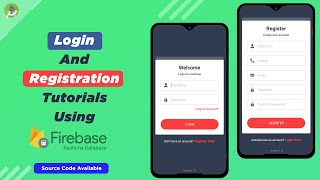
Take out your Android phones and try to count the number of applications that do not contain any login or authentication whatsoever. So you got it, right? There are very few applications that do not contain any login or authentication. When we start learning Android development, most of us must have actually created the basic login and login screen. So are we going to create a login and login screen here? No, but also yes 😉 Don’t worry, in this blog we will learn how to login and authenticate in our Android application but with the help of Firebase . This is how the flow of this blog looks like:
- Introduction to Firebase
- Login and authentication in Firebase
- Example – email login and registration
- Pros and cons of using Firebase
- Final Remarks
So let’s get started.
Reading: How to create a log in with firebase
Introduction to Firebase
Firebase was developed in 2011 to build and support mobile and web applications. Later, in 2014, it was acquired by Google. It offers you a number of possibilities that can be used for rapid development of your applications. So all you have to do is build applications quickly without having to think about managing the infrastructure. The following are Firebase’s products that can be used in any application:
- Cloud Firestore: This is a NoSQL database used for easy storing, synchronizing and queries data is used for job applications.
- ML Kit: It brings the power of machine learning to your applications.
- Authentication: Used to authenticate the users of your applications.
- Real Time Database: It is used to store and retrieve data in real time.
- Crashlytics: It provides you with the crash reports of your application.
- Test Labs: It tests your applications on the Google hosted devices.
- Performance Monitoring: Monitors the performance of your application every second.
- In-App Messages: It helps you to send in-app messages that help your users to interact with you.
- Google Analytics: It creates a report about your application. For example a report on the increase of users in the last month or active users etc.
- Remote Config: It helps you to change or add some features in your app without importing too an update.
Aside from the above Firebase products, some of the other Firebase products are cloud capabilities , hosting , cloud Storage , App Distribution , Predictions , A/B Testing , Cloud Messaging and Dynamic Links .
In this blog we will learn about the authentication part of Firebase.
Login and authentication in Firebase
Firebase authentication is used to authenticate the users of applications in a very simple way. Not only for the users but also for the developers it offers a very simple flow for the authentication and login process that is present in almost every application.
To authenticate your users, all you need to do is get the authentication credentials from the user and then pass those credentials to the Firebase Authentication SDK. These credentials can be an email password or a mobile phone number or any token from identity providers like Facebook, Google, Twitter, Github, etc. After passing the credentials, Firebase will validate the credentials and in return you will get a response telling you if the authentication succeeds or not.
Now let’s see how we can use Firebase login or authentication in our application.
Example – Login and registration via email
In this section of the blog we will learn how to login and register using email and password using Firebase. In this example we have four activities, viz. H. one for login, one for registration, one for password reset and one for our main activity. The following image describes the flow of activities:
In order to use Firebase authentication in our application, we need to update our project, i. H. the Android Studio project, connect to Firebase. Below are the steps to connect an Android project to Firebase:
Step 1: Open Android Studio and create a new project or open an existing project.
Step 2: Sign in to Android Studio with your email address. You can find the login button in the top right corner of Android Studio. (Remember the email id you used here)
Step 3: Open the Firebase website and log in. (use the same email id as in Android Studio for login)
Step 4: After login click Go to Console” which is present on the top right side of the website.
Step 5: Click “Add Project“.
Step 6: Enter the required project details and click Submit.
Step 7: After creating a project, you will see the following image of your project dashboard.
All Firebase services are displayed here and you can use any of them.
Step 8: In this blog, we are interested in the authentication part, so click the Authentication button and then switch to Login method ” Tab.
Step 9: In our example, we are logging in via email. So click the “Edit” button next to the “Email/Password” option and you will see the following screen.
See also: Dublin City
Step 10: Enable email/password login and click ” Save “. You are done with the Firebase website part.
Step 11: Return to your project in Android Studio and click Tools > Firebase > Authentication .
Step 12: We are almost done, now we have to add our project in Android Studio with the project we created in the Firebase. So click “Email and Password Authentication“. Here we have two options namely “Connect to Firebase” and “Add Firebase authentication to your app”. First we need to connect our project to Firebase, so click on the “Connect to Firebase” button.
Step 13: A list of projects associated with your email will appear and you need to select the project you created earlier on Firebase and then on “Connect to Firebase“.
Step 14: Now your project in Android Studio is connected to the existing one in Firebase add some dependencies, so click “Add Firebase authentication to your app” (this is the second option we found in step number 12). A dialog box will open. Click ” Accept Changes” and it will automatically add all dependencies to your project.
Phew! Finally, we’re done with all the steps required to deploy our Android Studio project with Firebase to connect for authentication. Now let’s get to the coding part.
As mentioned before, in our B example four activities:
- MainActivity: This is the main activity of the application. The user will be redirected to this activity if the user is successfully logged in or created a new account. In the MainActivity we have options to change the password and log out.
- LoginAcitivty: This activity is used to login the user to the application and after successful login transfer the user to MainActivity. It contains two EditText to take email and password from user. Two buttons for signup and signup (the signup button transfers the user to the SignupActivity) and a TextView that transfers the user to the ForgotPasswordActivity.
- SignupActivity: This activity is used to register the user in the application and after successful registration transfer the user to the main activity. It contains two EditText to take email and password from user for registration. Two buttons for login and login (the login button redirects the user to the login activity).
- ForgotPasswordActivity: This activity is used to send the Forgot Password link to the user to set a new password. The reset link will be sent to the user’s email id.
Register a user with email and password
To register a user with email and password, you must first create an instance of FirebaseAuth .
private latinit var auth: FirebaseAuth
Now initialize the FirebaseAuth instance in the onCreate() method.
auth = FirebaseAuth.getInstance()
To login a user with email and password we can use createUserWithEmailAndPassword() method. This method takes email and password as parameters, validates them, and then creates a new user.
auth.createUserWithEmailAndPassword(email, password).addOnCompleteListener(this, OnCompleteListener{ task -> if(task.isSuccessful){ Toast.makeText(this, “Successfully Registered”, Toast.LENGTH_LONG).show() valid intent = Intent(this, MainActivity::class.java) startActivity(intent) finish() }else { Toast.makeText(this, “Registration Failed”, Toast.LENGTH_LONG).show() } })
That’s it. Your user will be registered and you can see the registered user on the Firebase website in the Authentication section and then on the Users tab. If you try to register more than once with the same email address, you will receive an error message or, in simple words, your task will not be successful.
The full code of activity_signup.xml can be found here and SignupActivity.kt by here.
Register a user with email and password
In the previous section we saw how to register a user with email and password. Now we need to login the user by authenticating the email and password entered by the user.
First, declare an instance of FirebaseAuth.
private latinit var auth: FirebaseAuth
Now initialize the FirebaseAuth instance in the onCreate() method.
auth = FirebaseAuth.getInstance()
To sign in a user with email and password, we have a method called signInWithEmailAndPassword() . This method takes email and password as parameters, validates them, and then logs a user into your application if validation succeeds. The same code follows:
auth.signInWithEmailAndPassword(email, password).addOnCompleteListener(this, OnCompleteListener { task -> if(task.isSuccessful) { Toast.makeText(this, “Successfully Logged In”, Toast .LENGTH_LONG ).show() val intention = Intent(this, MainActivity::class.java) startActivity(intent) finish() }else { Toast.makeText(this, “Login Failed”, Toast.LENGTH_LONG).show() } } )
Full code of activity_login.xml can be found here and LoginActivity.kt from here .
Email the link to change the password
Sometimes the user forgot their password and in this case your application should provide a way to reset the password. Things get easier when you use Firebase. In Firebase you can use sendPasswordResetEmail() method to send password reset link to user and by clicking on this link user can set newer password.
First, create an instance of FirebaseAuth.
private latinit var auth: FirebaseAuth
Now initialize the FirebaseAuth instance in the onCreate() method.
auth = FirebaseAuth.getInstance()
See also: How to Design a Website with PowerPoint Templates?
After that you can use sendPasswordResetEmail() to send the reset email to the user. This method takes email as a parameter.
auth.sendPasswordResetEmail(email).addOnCompleteListener(this, OnCompleteListener { task -> if (task.isSuccessful) { Toast.makeText(this, “Reset the link sent to your email”, Toast.LENGTH_LONG) . show() } else { Toast.makeText(this, “Unable to send reset mail”, Toast.LENGTH_LONG) .show() } })
Check your email, you received a reset link 🙂
The full code of activity_forgot_password_activity.xml can be found here and ForgotPasswordActivity.kt here .
Check if a user is logged in or not
To check if a user is logged in or not we can use getCurrentUser() Method to get the status of a user.
if(auth.currentUser == null){ val intention = Intent(this, LoginActivity::class.java) startActivity(intent) finish() }else{ Toast.makeText(this, “Already logged in”, Toast .LENGTH_LONG).show() }
In the above code, if the user is logged in, a toast is shown, and if a user is not logged in, the LoginActivtiy is started.
Update Password
You can update a user’s password by using the updatePassword() method. This method takes the new password as a parameter and updates the password for you.
So create a FirebaseAuth instance and initialize the instance and after that use the updatePassword() method like this:
auth .currentUser ?.updatePassword(password) ?.addOnCompleteListener(this, OnCompleteListener { task -> if (task.isSuccessful) { Toast.makeText(this, “Password changed successfully”, Toast.LENGTH_LONG) .show() finish() } else { Toast.makeText(this, “Password not changed”, Toast.LENGTH_LONG) .show() } })
Similarly, you can change the email by updateEmail() method in the same way as in the case of updatePassword().
The full code of activity_update_password.xml can be found here and UpdatePassword.kt from here.
Log out the user
Now we are done with the login, login and password reset. Our next goal is to log out the user if they are already logged in. For this we have a method called signOut() . So just use this method to log out a user.
FirebaseAuth.getInstance().signOut()
The full code of activity_main.xml can be found here and MainActivity .kt from here .
Pros and cons of using Firebase
Some of the pros of using Firebase are:
- Easy integration into our project.
- There are so many functions available to perform complex operations.
- Real-time update.
- No server-side configuration required.
Some of the disadvantages of using Firebase are:
- The storage format is NoSQL. It uses JSON format.
- During authentication, you must ensure that the user logged into the app should not have access to the database.
Closing Notes
In this blog we learned how to use Firebase for login and authentication in our application. Firebase provides a number of methods to perform the authentication task in a very simple way. So, to perform any login or authentication task, you just need to use these methods. We’ve seen how we can use email and password to log into an application. Other ways of login and authentication are a phone number, Facebook, Google, Github, Twitter, etc.
Note: The project used in this blog is for example only. In general, you should use modularization concept to get better user experience and app performance. read here .
I hope you learned something new today.
Check out our Android tutorials here.
Share this blog with your fellow developers to spread your knowledge. You can read more blogs on Android on our blogging site.
Apply Now: MindOrks Android Online Course and Learn Advanced Android
Happy learning 🙂
Team MindOrks!
See also: How to Create a Distribution List in Outlook
.