Lets Develop an E-Commerce Application from Scratch Using Java and Spring
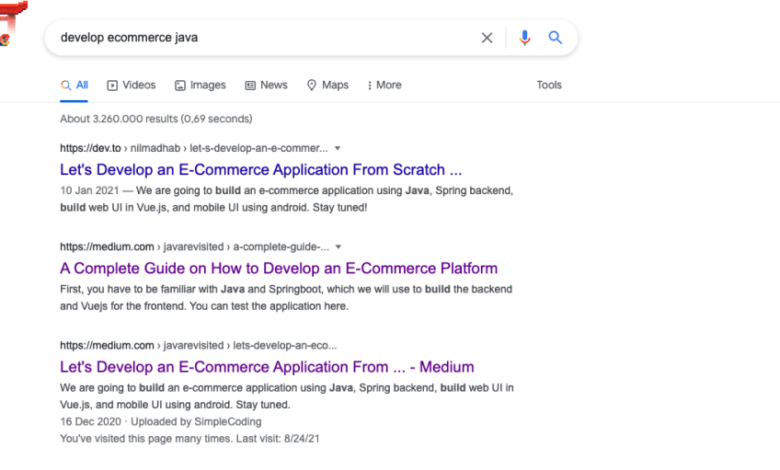
Project building, category and product API development
Motivation
In my opinion, the best way to learn programming is to create a real project that has some practical use has, this way the whole learning experience becomes quite exciting. Also, you can showcase your app in your portfolio, which can help you a lot if you’re looking to land a freelance job or an interview.
In this series of blogs, you’ll expand your development skills by learning how to building an e-commerce platform from scratch. First, you need to be familiar with Java and Spring Boot, which we will use to build the backend, and Vue.js, which we will use for the frontend.
Reading: How to create a ecommerce website using java
Note to readers:
Although I created the entire application and wrote a number of tutorials, which are very popular and rank high in Google results, which I’m very proud of (over 130,000 views on Medium alone)
I later realized that some parts in these tutorials are missing and some tutorials are no longer relevant are not. For example we used vanilla JS in some tutorials and also started developing an Android app which we later scrapped.
So this is my attempt to repeat the tutorials and delete some parts/ edit that are no longer relevant and create some tutorials that will cover the missing parts so that it will be very easy for users to follow the tutorials.
Video -Tutorial
Playlist
Frontend
Backend
Frontend Tutorial in Vue
Creating the project
-
First go to https://start.spring.io/ where we can create a new spring app and add dependencies
-
Choose maven, add Spring Data JPA and Spring Web dependencies
- Click Generate and load the . zip file i t and open it with IntelliJ idea
Project Structure
Main class
The project’s src/main/java folder contains a class that has a main method. It is the entry point for the application.
application.properties
In the src/main/resources folder there is a file called application.properties. This file will play an important role in telling the pen the configurations we make and how to create the object for us. In other words, it plays an important role in Inversion of Control (IoC).
pom.xml
In the project folder there is a file called pom.xml. In this file we add all the required dependencies.
Now the project structure looks like this:
You can check the project structure of the backend in the GitHub repository branch given below – GitHub — webtutsplus/ecommerce
Overview of our backend application
This Spring application lists the following important packages that you need to know before you start.
This is Spring architecture. The outside world calls the REST APIs that interact with the controller that interacts with the service. The service calls the repository.
The repository interacts with the database. We follow this pattern to make the code base maintainable instead of having spaghetti code which can be a nightmare in the long run.
Model / Entity
Model is the basic entity that has a direct relation to the structure of a table in the database. In other words, these models act as containers that hold similar and relative data that are used to transport that data from clients to the database. User Profile, Product and Category are some models in our backend application.
Repository
Repository is an interface that serves as a bridge between the database and the application.It carries the model data to and from the database. Each model has a unique repository for data transport.
Service
The service is the part of the architecture where the repository is instantiated and the business logic is applied. The client data arriving here is manipulated and sent to the database via the repository.
Controller
See also: How to create blog using PHP and MYSQL database?
The controller is the part of the architecture in which the client’s requests are first processed. It controls the processes to be run on the backend and the response that needs to be elicited from the clients. It interacts with the service, which in turn interacts with the repository, which in turn interacts with the database using models.
Journey of Data
Designing the Categories API
Once we have the basic structure ready, it’s time to create some products and categories for add our e-commerce store.
Take as an example, we can have a shoes category and have different types of shoes as a product. So a category can have many products, but each product belongs to a category.
Model
First we create a model, category. It will have four fields.
-
id
-
categoryName
-
description
-
imageUrl
We will also create a setter and getter for the four fields.
There will be a corresponding table category in the database
We use the @NotBlank annotation for the category. For that we need to include the following dependency in the pom.xml file.
Repository
Now let’s create a repository Categoryrepository.java that extends JpaRepository.
This will have a findByCategoryName method.
Service
Now we will create a CategoryService file that is responsible for creating, updating or retrieving repositories.
The category repository is built in methods findAll(), save() as an extension of JpaRepository
Controller
We will create a helper class ApiResponse.java that will be used to return a response for the APIs.
Now we will create the controller that will contain all the APIs
We will create 3 APIs for the category
-
create
-
update
-
list all categories
We will also add boast for easy code testing. We also need to add these dependencies in pom.xml file for swagger and h2 in memory database. However, you are free to choose another database of your choice.
We also need to modify our application.properties file by adding the lines
Now run the code and open http://localhost:8080/ swagger-ui .html page. We will see this screen
Demo
Let’s create a category monitor , with this request text. (Note: We don’t need to pass an ID here, it will be generated automatically.)
You will get the following Answer:
See also: A Beginners Guide to learn web scraping with python!
Let’s click the Get API now
We get the following response:
Enable CORS
We will add the webconfig.java file to allow our frontend to access the API.
Hooray! We can now play with the APIs and create some new categories, update and get all categories.
Designing the Product API
Now we have some categories, it’s time to they create the product APIs. First we create the model, then we create the repository, then we create the service and at the end we create the controller and test it.
Model
The product has an ID, a Names, an image URL, a price, a description, and a foreign key for the category since each product belongs to a category.
Repository
Next we create a ProductRepository file. java in the repository package that just extends JpaRepository. If we need some methods, we will add them later
Service
Now we can create the service class. Create a ProductService.java file in the service directory. It will have an automatically wired ProductRepository.
DTO Concept#
Before we create a product, we need to understand what a DTO (Data Transfer Object) is
Martin Fowler came up with the concept of a Data Transfer Object (DTO) as an object that transports data between processes.
In the Controller category, we used the model directly as the request body, but this is impractical in many cases. We need to create another object because
-
sometimes the model might need to be changed and we don’t want to change the API for backwards compatibility
-
We cannot use the model as request body if it has a relation to another model.
So quickly we will create a package dto and inside the package we create another package product and there we create our ProductDto.java class which will have the following attributes
We also pass categoryId as we need this to associate a product with a category.
Controller
Now that we have the productDto ready, it is now time to create the ProductController.java class
It will automatically create ProductService and CategoryService wire
Create a new product API
We get the category ID and product details from the request body.
First we check if the category id is valid or return “Category is invalid”. Error.
Then we create a product by calling method, productService.addProduct, which takes productDto and category as arguments.
Full code is available in GitHub repository given below – GitHub — webtutsplus/ecommerce at product-apis
And this marks the end of this tutorial. But wait! The tutorial series continues to create the UI using Vue.js for the backend application developed above. Until then, stay tuned!
Have fun learning
Proceed to the next tutorial where we will use the API to build a frontend to create with vue.js
See also: How to create a 3D logo
.