Creating A Shopping Cart With HTML5 Web Storage
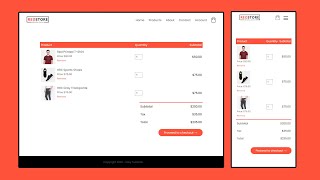
- Read in 12 minutes
- Ecommerce, HTML5
- Share to Twitter, LinkedIn
With the advent of HTML5 Many websites have been able to replace JavaScript plugins and codes with simple, more efficient HTML codes such as audio, video, geolocation, etc. HTML5 tags made developers’ work much easier while improving page load time and website performance. HTML5 web storage in particular was a game changer as it allowed users’ browsers to store user data without using a server. So, creating web storage enabled front-end developers to do more on their website without knowing or using server-side coding or database.
Online e-commerce websites mostly use server-side languages like PHP to store user data and pass it from one site to another. With JavaScript backend frameworks like Node.js, we can achieve the same goal. However, in this tutorial, we will show you step-by-step how to create a shopping cart using HTML5 and a little bit smaller JavaScript code. Other uses of the techniques in this tutorial would be to store user preferences, user’s favorite content, wish lists, and user settings like name and password on websites and native mobile apps without using a database.
Reading: How to create a shopping car for a website
Lots of high traffic -Websites rely on complex techniques such as server clustering, DNS load balancers, client-side and server-side caching, distributed databases, and microservices to optimize performance and availability. In fact, the biggest challenge for dynamic websites is getting data from a database and using a server-side language like PHP to process it. However, remote database storage should only be used for essential website content such as articles and user credentials. Features such as user preferences may be stored in the user’s browser in a manner similar to cookies. Likewise, when building a native mobile app, you can use HTML5 web storage in conjunction with a local database to increase the speed of your app. Therefore, as front-end developers, we need to explore ways to leverage the power of HTML5 web storage in our applications in the early stages of development.
I was part of a development team for a large social website, and we made heavy use of HTML5 web storage. For example, when a user logs in, we store the hashed user ID in an HTML5 session and use it to authenticate the user on protected pages. We also use this feature to store any new push notifications – like new chat messages, website messages and new feeds – and push them from one page to another. When a social site gets heavy traffic, relying entirely on the server for load balancing may not work, requiring you to identify tasks and data that can be processed by the user’s browser instead of your servers.
Project background
A shopping cart allows a website visitor to view product pages and add items to their shopping cart. The visitor can review all of their items and update their shopping cart (e.g. to add or remove items). In order to achieve this, the website needs to store the visitor’s data and pass it from one page to another until the visitor goes to the checkout page and makes a purchase. Storing data can be done through a server-side or a client-side language. With a server-side language, the server bears the weight of data storage, while with a client-side language, the visitor’s computer (desktop, tablet, or smartphone) stores and processes the data. Each approach has its pros and cons. In this tutorial we will focus on a simple client-side approach, based on HTML5 and JavaScript.
Note: Basic knowledge of HTML5, CSS and JavaScript is required to follow this tutorial is required.
Project Files
Click here to download the project source files. You can also watch a live demo.
HTML5 web storage overview
HTML5 web storage allows web applications to store values locally in the browser that can persist throughout the browser session , just like cookies. Unlike cookies, which must be sent with every HTTP request, web storage data is never transmitted to the server. Therefore, web storage outperforms cookies in web performance. Additionally, cookies only allow you to store 4KB of data per domain, while web storage allows at least 5MB per domain. Web memory works like a simple array that maps keys to values and comes in two types:
- Session memoryThis is where data is stored in a browser session where it becomes available until the browser or browser tab is closed. Pop-up windows opened from the same window can show session storage, as can iframes within the same window. However, multiple windows from the same origin (URL) cannot see each other’s session storage.
- Local storageHere, data is stored in the web browser without an expiration date. The data is available to all windows with the same origin (domain), even if the browser or the browser tabs are opened or closed again.
Both types of storage are currently supported by all common web browsers .Remember that you cannot pass save data from one browser to another, even if both browsers visit the same domain.
Create a simple shopping cart
To create our shopping cart, We’ll first create an HTML page with a simple shopping cart to display items and a simple form to add or edit the cart. Then we add HTML web storage followed by JavaScript coding. Although we use HTML5 local storage tags, all steps are identical to HTML5 canister storage and can be applied to HTML5 canister tags. Finally, for those who want to use jQuery, let’s go through some jQuery code as an alternative to JavaScript code.
Add HTML5 Local Storage to Cart
Our HTML page is a simple page with tags for external JavaScript and CSS referenced in the header.
Below is the HTML content that appears in the body of the page:
Add JavaScript to Cart
We create and call the JavaScript function doShowAll() in the onload() event to check the browser support and dynamically create the table that shows the memory name-value pair.
See also: Recruiting Column: How to build an effective college recruiting résumé
Alternatively, you can use the JavaScript onload event by adding the following to the JavaScript code:
window.load=doShowAll();
Or use this for jQuery:
$( window ).load(function() { doShowAll(); });
In the CheckBrowser() function, we want to check whether the browser supports HTML5 saving. Note that this step may not be necessary as most modern web browsers support it.
When the CheckBrowser() function inside doShowAll() first evaluates browser support, it dynamically creates the table for the shopping list when loading the page. You can iterate over the keys (property names) of key-value pairs stored in local storage within a JavaScript loop, as shown below. Based on the memory value, this method dynamically populates the table to show the key-value pair stored in local memory.
Note: Either you or your framework have a preferred method for Creating new DOM nodes and handling events. To keep things clean and focused, our example uses .innerHTML and inline event handlers, although we would normally avoid this in production code.
Tip: If you want to use you jQuery to bind data, you can just replace document.getElementById(‘list’).innerHTML = list; with $(‘#list’).html()=list;.
Run the cart and test
In the previous two sections, we added code to the HTML header, and we have added HTML to cart form and shopping cart. We’ve also created a JavaScript function to check browser support and populate the cart with the items in the cart. When filling the shopping cart items, the JavaScript retrieves values from HTML web storage instead of a database. In this part, we will show you how to inject the data into the HTML5 storage engine. That is, we use HTML5 local storage in conjunction with JavaScript to add new items to the cart and edit an existing item in the cart.
Note: I have Added tips sections below to view the jQuery code as an alternative to the JavaScript codes.
We will create a separate HTML div element to capture user input and submissions. We put the appropriate JavaScript function in the onClick event for the buttons.
You can set properties on the localStorage object similar to a regular JavaScript object. Here’s an example of how we can set the local storage property myProperty to the value myValue:
localStorage.myProperty=”myValue”;
You can delete the local storage property as follows:
delete localStorage.myProperty;
Alternatively, you can use the following methods to access local storage:
localStorage.setItem(‘propertyName’,’value’); localStorage.getItem(‘propertyName’); localStorage.removeItem(‘propertyName’);
To save the key-value pair, retrieve the value of the appropriate JavaScript object and call the setItem method:
function SaveItem() { var name = document.forms.ShoppingList. name.value; var data = document.forms.ShoppingList.data.value; localStorage.setItem(name, data); doShowAll(); }
See also: 7 Ways to Write Better Recipes as a Food Blogger
Below is the jQuery alternative for the SaveItem function. First add an ID to the form inputs:
Then select the form inputs by ID and get their values. As you can see below, it’s much simpler than JavaScript:
function SaveItem() { var name = $(“#name”).val(); var data = $(“#data”).val(); localStorage.setItem(name, data); doShowAll(); }
To update an item in the shopping cart, you must first check whether the key of that item already exists in the local store, and then update its value, as shown below:
You can see the below jQuery alternative.
We use the removeItem method to delete an item from memory.
Tip: Similar to the previous two functions, you can use jQuery selectors in the RemoveItem function.
There is another method for the local Storage that allows you to clear all local storage. We call the ClearAll() function in the onClick event of the “Clear” button:
We use the clear method to clear local storage as shown below:
function ClearAll() { localStorage.clear(); doShowAll(); }
Session Storage
The sessionStorage object works the same as localStorage. You can replace the example above with the sessionStorage object to expire the data after a session. As soon as the user closes the browser window, the memory is cleared. In short, the APIs for localStorage and sessionStorage are the same, so you can use the following methods:
- setItem(key, value)
- getItem(key)
- removeItem(key)
- clear()
- key(index)
- length
Shopping carts With Arrays and Objects
Because HTML5 web storage only supports storing individual names and values, you must use JSON or some other method to convert your arrays or objects to a single string. You may need an array or object if you have a category and subcategories of items or if you have a shopping cart with multiple data like customer information, item information, etc. All you have to do is implode your array or object elements into a string to store them in web storage, and then unarray (or split) them back into an array to display on another page. Let’s walk through a simple example of a shopping cart that contains three sets of information: customer information, item information, and custom mailing address. First we use JSON.stringify to convert the object to a string. Then we use JSON.parse to undo it.
Note: Remember that the key name should be unique for each domain.
Summary
In this tutorial we have learned step by step how to create a shopping cart using HTML5 web storage and JavaScript. We’ve seen how to use jQuery as an alternative to JavaScript. We also discussed JSON functions like Stringify and Parse to handle arrays and objects in a shopping cart. You can build on this tutorial by adding more features, e.g. B. Adding product categories and subcategories while storing data in a multidimensional JavaScript array. In addition, you can replace all JavaScript code with jQuery.
We’ve seen what else developers can do with HTML5 web storage and what other functionality they can add to their websites. For example, you can use this tutorial to store user settings, preferred content, wish lists, and user settings like names and passwords on websites and native mobile apps without using a database.
Finally, here are some points to consider at implementing HTML5 web storage:
See also: How to Create Social Media Buttons For Every Major Network
- Some users may have privacy settings that prevent the browser from storing data.
- Some users may be using their browser incognito mode .
- Be aware of some security issues such as DNS spoofing attacks, cross-directory attacks and compromise of sensitive data.
Further Reading
- ” Browser Storage Limits And Eviction Criteria”, MDN Web Docs, Mozilla
- “Web Storage”, HTML Living Standard,
- “This Week In HTML 5”, The WHATWG Blog
- li>
.