How to Create a Simple Web App Using JavaScript
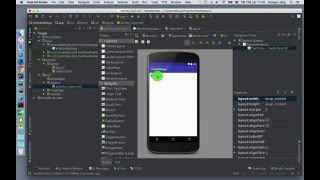
PSA: JavaScript (JS) is dangerously addictive. So addicted that I develop random web apps in my spare time to entertain myself and of course practice some JS coding skills. JavaScript, like any foreign language, may seem daunting at first (who is it anyway? and why doesn’t it like me?). But remember:
We can start building a simple, interactive web app using JS. Also, let’s aim to accomplish this in about 20 lines of code. Ready? Here we go!
Reading: How to create an app using javascript
What we’re building
Let’s build a web app that will serve as a daily self-motivator and tool to brighten your mood. I got it. An app that shows you a random Kanye quote along with the option of a random cat photo. Awesome!
To implement this idea, we can use 2 different APIs (Application Programming Interface) to help with the backend, 1) Kanye.Rest and 2) the Cat API
https://kanye.rest
The App
Open your favorite text editor (I use VS Code) and create a repository with 2 files: index.js and index.html . The index.js file is where all your JS code will go. The index.html file contains the structure and format of your web page.
Because we’re building a simple web application, we won’t use any advanced CSS formatting or styling here. The main structure of your HTML file looks like this:
Make sure the HTML file contains this line of code:
The “defer” tells the browser to wait for the page to load to execute the JavaScript.
Next we should create a div, in which the Kanye quote will be placed. Let’s give this div an ID called “quotes”. That way we can easily find that div with that specific ID when we need it.
Pro Tip: It’s always best to add IDs to your important HTML tags, especially to the ones you know you need to reach into your code and call. It’s easier to find HTML elements by “ID” than by class name because IDs MUST be unique!
See also: How to Create an Online Magazine With WordPress (In 3 Steps)
We should create a button that when clicked will show a random cat photo in the browser. The button has the id “give-cat”.
Lastly, create a div with the id “cat-pic” that contains the cat photo.
Now , let’s go to the file index.js and start programming JS!
Use the Kanye API to get Kanye quotes
The first step we should do is to add the div to find where the quote will be placed and declare it as a variable. We can do this by typing:
let quotesDiv = document.getElementById(‘quotes’)
To retrieve data from the Kanye and Cat APIs we need to use the retrieve function. For this first part, we’ll send a GET request to the Kanye API.
The fetch returns an object in response to the request. We call the .json() method on the response to parse the response body as JSON.
Next, we run another .then(). Here we get the JSON object that we can finally use to manipulate the DOM! We can name this new JSON object Quote.
Remember the variable quotesDiv we declared earlier? We need to use it here now. In order for us to append this quote to the DOM, we can call the innerHTML property of quotesDiv.
If we look at what the JSON object looks like, we can see that the quote is also a key.
So we write:
The whole block of code should look like this:
See also: How to Launch an NFT Minting Page – Full Walkthrough
Let’s see how our DOM looks now!
Impressive! On to the next delivery!
Use the Cat API to get cat images
Now we need to add onclick functionality to the button. Grab the button by finding it by ID and declare it as a variable called catButton.
let catButton = document.getElementById(‘give-cat’)
Next we need to give the button an event -Add listener. The event listener takes two arguments: 1) the event 2) a callback function to handle the event.
catButton.addEventListener(“click”, evt => {
Before we make the call, let’s determine the part of the DOM where the cat photo goes Find the div with the id “cat-pic” and declare it as a variable.
let catDiv = document.getElementById(‘cat-pic’)
Well, we’re making a GET request to the Cat API, in the last .then() we need to do a forEach method on the JSON response since it’s an array.
We call the innerHTML property on catDiv . Let’s add a nice header and image tag for the image. “URL” is a key in the response object, so we need to use it here to display the image.
Moment of truth! Let’s see how our last page looks!
Woohoo! It works! 🙌🎊 .
Let’s go back to our code and one last take a look at it en.
It seems that we also achieved our goal of completing this task in about 20 lines of code!
The more practice you get with JavaScr ipt, the more fluent you will become in the language (obvs). Try out “fun APIs” to use in your next creative project. Who knows? You might end up creating the next Instagram.
See also: Create a drop-down list
.