How to build a full-page website in Angular
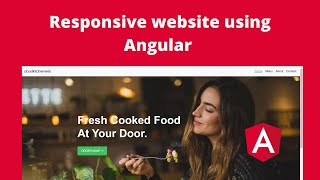
The latest version of Angular is often seen as a framework that originated from the enterprise side of the tracks and generally enjoys the company of line-of-business applications. While it’s true that Angular has indeed evolved from a framework to a platform that supports all types of applications, there are many truly amazing features available to developers to create immersive experiences for their users.
This is the first part of a series on how to create a full page animated website in Angular. We will start by building a full page website and then animate it in the following episode.
Reading: How to create a website using angular 4
In this tutorial we will focus primarily on the Angular parts and refrain from talking about the HTML and CSS parts that are not directly related to the construction of the application. Please download the source code (opens in new tab) to participate. Not sold on Angular? Find the perfect website builder here (and best prices below). However you create your website, you need decent web hosting and cloud storage.
01. Building a project
There are many moving parts that go into a non-trivial web application. What dependencies does your application have? How do you want to run it locally? How do you want to test it? How will you bundle your assets?
Fortunately, the complex process of assembling these elements is handled in the @angular/cli. With just a few commands from our terminal, we’ll have a fully functional Angular application to work with.
The first step to working with the CLI is installation. To do this, use the following command:
npm install -g @angular/cli
Once the CLI is installed, we can navigate from the command line to the folder where we want to install our project. From there we can run ng new with the name of our project. This will create a folder with the same name that we will navigate to after the project is complete.
cd ng new angle-animations-site cd angle-animations-site
See also: How to Create a Blog Content Strategy – What 4 Years of Experience Have Taught Me
And that’s it it! Our Angular application is up and running. You can start your application with either npm start or ng serve. I prefer using npm start because it’s more conventional and allows me to add additional commands. You can then navigate to http://localhost:4200 to see how the application is running.
02. Add Animations and Angular Material
Since we like beautiful things, we will make some small additions to our application by adding @angular/animations and and install @angular/material packages:
npm i -save @angular/material @angular/animations
We can inform Angular about these dependencies by adding them to our app. Add module.ts file. We’ll be using Angular Material’s buttons, cards, and toolbars, so we’ll import their respective modules, as well as the BrowserAnimationsModule.
// app/app.module.ts … import { MdButtonModule , MdCardModule, MdToolbarModule } from ‘@angular/material’; import { BrowserAnimationsModule } from ‘@angular/platform-browser/animations’;
We can then add them to the imports array with our NgModule declaration.
// app/app.module.ts … import {MdButtonModule, MdCardModule, MdToolbarModule} from ‘@angular/material’; import { BrowserAnimationsModule } from ‘@angular/platform-browser/animations’; @NgModule({ … imports: [ … BrowserAnimationsModule, MdToolbarModule, MdButtonModule, MdCardModule ], … })
And as a final addition, we will import the indigo pink theme into our styles. css file.
/* styles.css */ @import ‘~@angular/material/prebuilt-themes/indigo-pink.css’;
Up to this point we’ve met quite focused on that when setting up the application so we could start development. These commands may feel clunky at first, but once you get used to them, you’ll find that it only takes a few minutes to have a fully integrated environment with all the bells and whistles we need to build a cute website.
03. Introduce a page component
Since we are building a website with Angular, we need to introduce a mechanism for displaying our pages. In Angular, the atomic building block of an application is the component. By architecting our application with well-defined, encapsulated components, we are able to easily reuse functions and create new functions by introducing new components.
The CLI comes with direct generator support, and that’s what we use to create our page component. We can generate our page component by running the following command (the g command is an abbreviation of generate).
ng g components page
See also: How to Start a YouTube Channel for Your Small Business
Note: I recommend taking the time to learn how to hand build the main Angular parts until you build your muscle memory. Only when you really understand what is happening should you optimize your workflow with generators.
The CLI generates a folder in the src directory called page with an HTML, CSS and Typescript file and a specification file. In our page.component.ts file we have the basic structure of a component. Our component references our template and style files in the @Component metadata and has hidden our constructor and ngOnit methods.
// app/page/page.component.ts import {Component, OnInit} from ‘@angular/core’; @Component({ selector: ‘app-page’, templateUrl: ‘./page.component.html’, styleUrls: [‘./page.component.css’] }) export class PageComponent implements OnInit { constructor() { } ngOnInit() { } }
Apart from generating our component, the CLI also modifies our app.module.ts to include a PageComponent entry in our Declarations array. This means that our page component is now available throughout the module.
// app/app.module.ts @NgModule({ declarations: [ AppComponent, PageComponent ], … })
As a sanity check, we can we jump into our app.component.html file and add at the bottom. Note that the element tag we used corresponds to the selector property defined in our @Component metadata.
{{title}}
04 . Build your page component
Once our page component is alive and well, we can build it to look like a real web page. We will introduce a Page object with Title, Subtitle, Content and Image properties.
// app/page/page.component.ts export class PageComponent implements OnInit { page = { title: ‘Home’, subtitle: ‘Welcome Home!’, content: ‘Some home content.’, image : ‘assets/bg00.jpg’ }; constructor() { } ngOnInit() { } }
We will update our template to fit the Page object. There is an image element that eventually expands to fill the entire browser window. We will also add an Angular Material map component to which we will bind the rest of our Pages object.
{{page.title}}
{{page.subtitle}} {{page.content} }
Our page component looks a lot better! Our next step is to add the ability to have multiple pages and navigate between them.
Page 2: How to create multiple pages
See also: How Much Does It Cost to Build a Website for a Small Business in 2023?
.